Here in the last in our series on making a sundial using OpenSCAD we need to finish the dial by draw the hour numbers. We know that the hour angle as measured at the foot of the gnomon is:
tan(theta) = sin(lat)*sin(HA)
where HA is the hour angle from noon (15 deg for each hour)
lat is the latitude
theta is the hour line
theta = atan2(sin(lat)*sin(HA), cos(HA))
ao = 1
bo = -2*goffset*cos(theta)
co = goffset^2 - crad^2
The idea is to set up a loop for drawing the hour numbers. Let's say that we want to put down the numbers 5(am) to 7(pm). On a 24 hour scale, that's H = 5:19. Meanwhile that hour angle referenced to the 12-noon hour is HA = 15*(H-12) degrees.
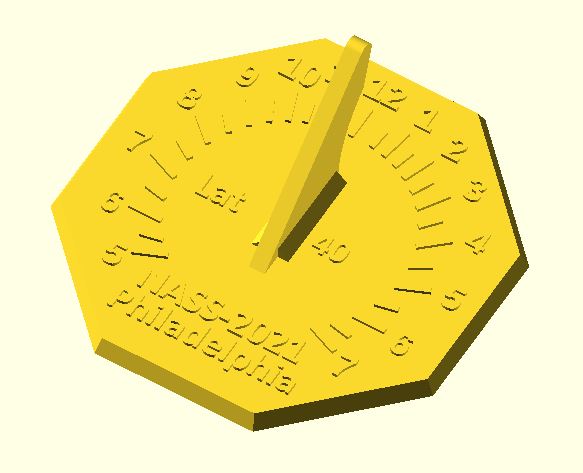
//text of one hour number
if (H>12) {
txt3 = str(H-12);
}else{
txt3 = str(H)
}
//classic hour line
theta = atan2(sin(latitude)*sin(HA),cos(HA));
if H {
wo = gnomon_width;
}else{
wo = -gnomon_width;
}
//this is a distance goffset from the dial center. We need to use the quadratic
//equation to get the distance from the gnomon foot to a circular arc (chapter ring)
//of radius crad where our numbers will be placed...
push = 1.15; //tweak to move the number a bit further out on the dial face
ao = 1;
bo = -2*goffset*cos(theta);
co = goffset*goffset - crad*crad;
zo = push*abs((-bo - sqrt(bo*bo-4*co))/(2*ao));
//translate this to the dial x,y coordinate system
xo = zo*sin(theta) + wo/2; //remember that wo may be positive or negative
yo = zo*cos(theta) + goffset; //goffset is a negative number since moved gnomon south of center
//print the number
translate([xo,yo,dial_thickness]) //raise the number to sit at the top of the dial face thickness
linear_extrude(height = ho) //ho is the thickness (height) of our number
text(txt3, size = 4.5, halign = "center",font = str("Angsana:style=Bold"), $fn = 16);
}
The final OpenSCAD code to create this simple horizontal sundial is attached. Download it and have fun.